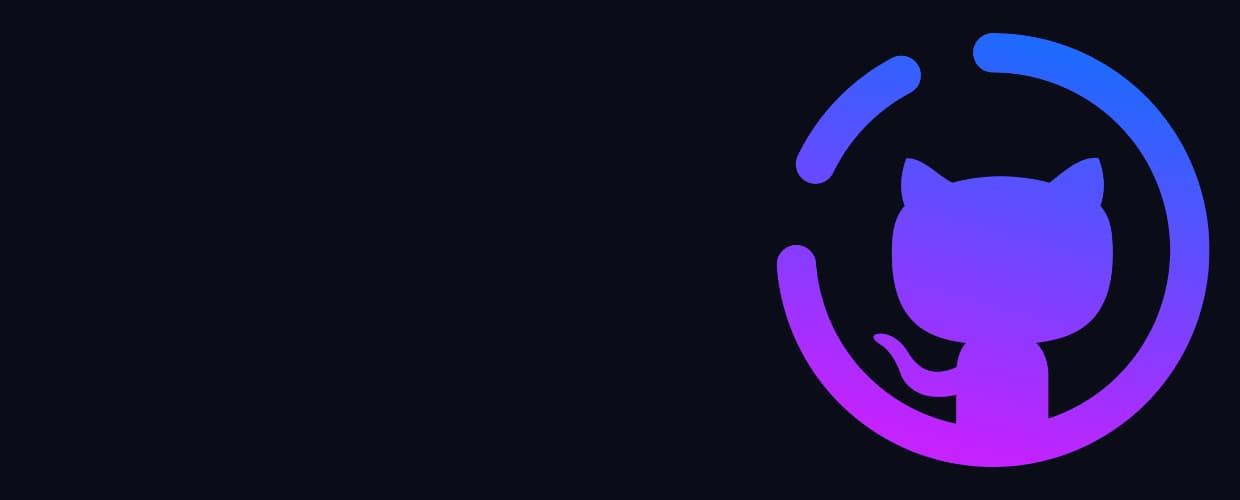
Github API - Integration
Integrating GitHub API into your project using Python
GitHub's API allows developers to programmatically interact with repositories, issues, and pull requests. This tutorial covers integrating the GitHub API into your Python project, including authentication, making requests, and handling responses.
Lets Get Started
Generate Personal Access Token:
- Go to your GitHub account settings.
- Navigate to Developer settings -> Personal access tokens -> Tokens (classic).
- Click on "Generate new token" -> Generate new token (classic).
- Give your token a description and select the appropriate scopes (permissions).
- Click
"Generate token"
and copy the generated token.
Note: Treat this token like a password and keep it secure.
I have selected the following read permissions only to view them public on my portfolio.
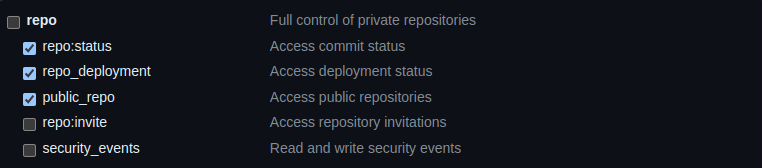


Save your github username and token inside .env
file.
About Github REST API
You can use GitHub's API to build scripts and applications that automate processes, integrate with GitHub, and extend GitHub. For example, you could use the API to triage issues, build an analytics dashboard, or manage releases.
In this blog we are going to integrate the only API which can we show public like repositories, your profile details and more.
Pinned repositories
Lets fetch our pinned repositories
first
Replace the " quotations with ` backticks and also replace greater than and less than signs with $ querly braces
Save response inside a state to render to on jsx element.
Profile details
Now fetch github profile details
Replace the " quotations with ` backticks and also replace greater than and less than signs with $ querly braces
Save response inside a state to render to on jsx element.
Contributions
Last but not least lets fetch github contributions
Replace the " quotations with ` backticks and also replace greater than and less than signs with $ querly braces
Here I'm using graphql query which I will explain below the code snippet.
The query retrieves data about the user's name and contributions, including their total contributions and contribution calendar details.
Save response inside a state to render to on jsx element.
Overview of GraphQL
- GraphQL is a query language for APIs developed by Facebook. It allows clients to request only the data they need, in a single query, from a GraphQL server.
- Unlike REST APIs, where clients often receive fixed data structures, GraphQL APIs allow clients to specify the shape and structure of the data they require.
- GraphQL provides a more efficient and flexible way to retrieve and manipulate data, making it particularly useful for applications with complex data requirements or multiple data sources.
Why GraphQL is Used Here
- In this code, GraphQL is used to fetch contribution data from the GitHub API.
- GraphQL allows the query to be tailored precisely to the data needed, reducing over-fetching and under-fetching of data compared to traditional REST APIs.
- By using GraphQL, the code can efficiently retrieve specific contribution details for the user, including their total contributions and contribution calendar, in a single request.
- The form of data we are retrieving will make it easier to render it on a chart.
Destructuring the response
Lets destructure our data recieved from our pinnded, profile and contributions requests
linksData
Congrats
You have successfuly implemented the diffucult part.
When it comes to HTML and styling, I trust your expertise to create something truly user-friendly and amazing. Feel free to use your creativity and the information you have to make it shine!
Look what I have came up with
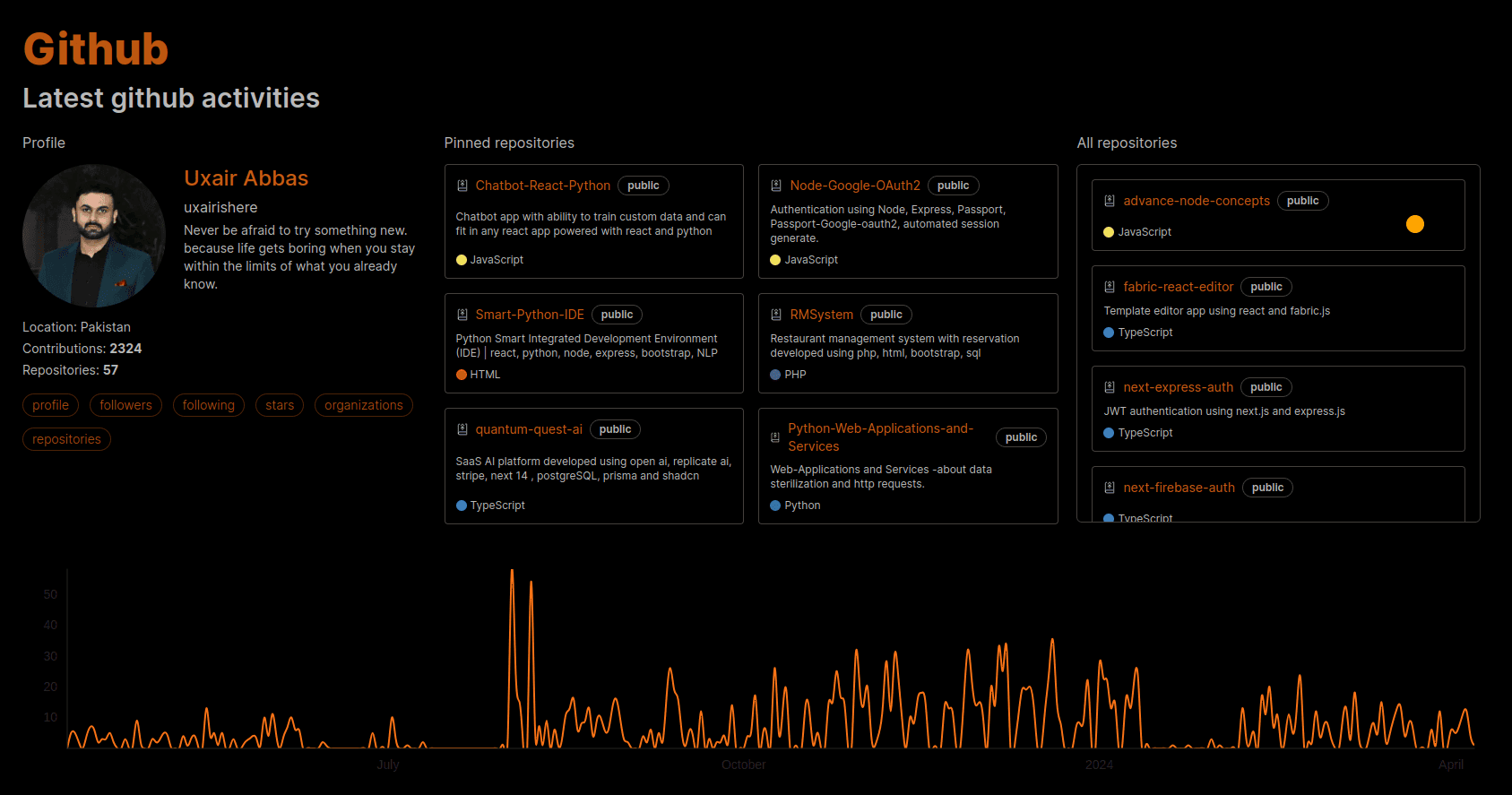